Writing Your First Blender Script
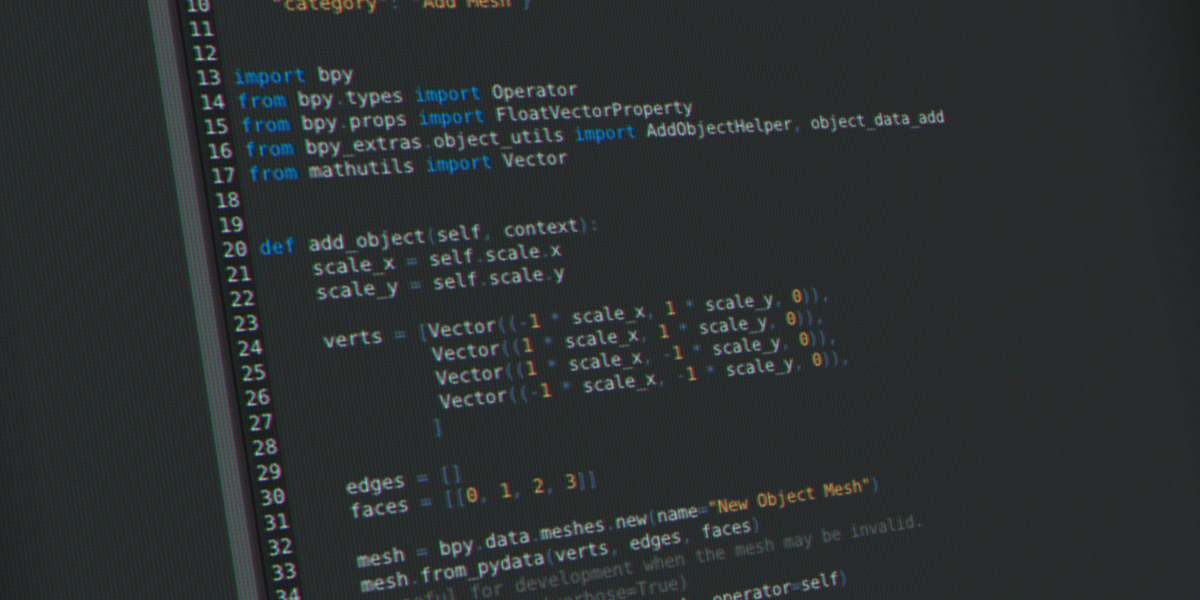
Anyone that has worked with Blender for more than a few hours will likely have used an add-on. Add-ons primary purpose is to alter, improve, or otherwise change your workflow in Blender. There are thousands of add-ons available, for all versions of Blender. However, sometimes you just need something custom or you can't find an add-on to solve a present problem. Wouldn't it be nice if you could create your own add-ons? Well, you can. It's not even hard.
Learn how to create your own add-on with help from Bassam Kurdali
Identify a problem in your workflow
The first step in creating any kind of add-on is finding a problem to solve. This is not, generally, a case of looking for a problem, but rather identifying a hindrance or nag in your everyday workflow that could be improved. For example, one of the first add-ons I wrote was Quick Tools. When I started building Quick Tools I knew next to nothing about building add-ons for Blender, but there were kinks in my workflow and they bothered me. So I decided to take the plunge and fix them myself, which required I learn the basics of Python scripting.
Learning the basics of scripting in Blender
Scripting. Programming. Coding. These are all words that practically strike fear into the hearts of many artists. Honestly, though, scripting is not hard to learn and it will empower you, as an artist, more than any other tool at your disposal. Why? Because it better enables you to work how you want to work, not how other people think you might work. Programming comes in many forms, but for Blender, it's mostly Python scripting. Python is a programming language that values readability and simplicity, making it perfect for those of us that are not hard-core programmers. Blender comes with a Python API, which gives access to many of the same tools and abilities that make up the regular internal tools, allowing people to create their own tools. As a real-world example, check out the script below. It's just a few lines but can easily save you a lot of time if you need to add Subdivision Surface modifiers to a lot of objects. Go ahead, try it! Just copy the code below and paste it into Blender's text editor and press ALT+P. Any selected mesh object will get a SubD modifier.
# Bring in Blender's Python API import bpy # Create a list of all the selected objects selected = bpy.context.selected_objects # Loop through all selected objects and add a modifier to each. for obj in selected: bpy.context.scene.objects.active = obj bpy.ops.object.modifier_add(type='SUBSURF')
See? It's really not hard. Even simple scripts like the one above can make a big difference in your workflow. Learning Python is not hard. Want to get started in less than an hour? Join CG Cookie and learn from Bassam Kurdali's Introduction to Python Scripting for Blender Artists. Bassam is one of the best. He was the director of Elephants Dream and is now working on the Tube Project, known as Wires for Empathy.
Start small, solve the problem with a script
A common pitfall for anyone learning a new skill, but particularly programming, is starting too large. Expecting to learn programming and to build something like RetopoFlow or MotionTool in the same week is simply not realistic. However, starting small is very realistic and the implications can still be dramatic. As an example, check out my tutorial on Automating Tasks with Python in Blender. Doing these kinds of small scripts can greatly speed up and improve many of your day to day tasks, allowing you to work faster and more efficiently. If you're a working professional then this is a big deal. As they say, time is money.
Improve the script
After you've created your first script just keep iterating. It doesn't need to be perfect the first time. Messy code that works is better than clean code that fails. Continue making incremental improvements over time. Not only will this make your script(s) better, but it's also a great learning experience as it brings about many opportunities to learn things you might otherwise ignore. Taking our initial script above, we can improve it a bit by making a reusable function for adding modifiers and by checking if any SubSurf modifiers already exist on the object:
import bpy # Create a list of all the selected objects selected = bpy.context.selected_objects # Make a reusuable modifier add function def addMod(modifier): bpy.ops.object.modifier_add(type=modifier) # Iterate through all selected objects for obj in selected: bpy.context.scene.objects.active = obj # Check if modifiers exist if not obj.modifiers: addMod('SUBSURF') else: for mod in obj.modifiers: if mod.type == 'SUBSURF': break else: addMod('SUBSURF')
Note: this code is not very concise, it could be made shorter, with less conditional statements, but I intentionally kept it like this for the sake of beginner readability.
To make things easy to maintain, you can take it a step further by learning the basics of Git for version control. Perhaps you can even start uploading your scripts to a Github account and sharing them around.
Develop the script into an add-on
Once your script is working, if it's something that you'll use more then once it may be worth turning it into an add-on. This simply makes it into an installable script that's easy to turn on and off. Doing so also makes it much easier to share with the community. You can learn how to transform a script into an add-on in Bassam's course on Creating a Custom Rig UI. The only question now, is what will you create? If you've already written some scripts, or are in the process of doing so, share them below in the comments! I'd love to see what you're doing.